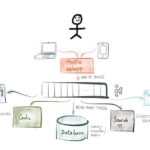
Event Sourcing and Event-Driven Architecture: Complementary Techniques for Building Robust Software Systems
August 2, 2023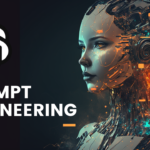
Prompt Engineering; Effective interaction with AI models
August 22, 2023Event-Driven Serverless Microservices Web Application Engineering Introduction
Welcome to today's comprehensive lecture on "Event-Driven Serverless Microservices Web Application Engineering." In this session, we will delve deep into the intricacies of constructing modern web applications using a microservices architecture. Our focus will be on the event-driven design philosophy and the seamless integration of serverless computing. Throughout our lecture, we will provide Node.js code samples and valuable references to enhance your understanding.
Section 1: Understanding Microservices Architecture
Microservices architecture is a paradigm where a complex application is broken down into smaller, independent services. Each service operates as a self-contained unit, communicating with others through well-defined APIs. This architectural style offers several advantages, including enhanced scalability, improved fault isolation, faster development cycles, and better maintainability.
References:
Martin Fowler's blog on Microservices: https://martinfowler.com/microservices/
Kubernetes Documentation: https://kubernetes.io/docs/home/
Section 2: Event-Driven Architecture
2.1: Core Concepts of Event-Driven Architecture
At the heart of an event-driven architecture are events, which represent significant occurrences in the system. Events can range from user interactions to data changes or external triggers. These events are generated by components and propagate to interested parties through event brokers.
2.2: Benefits of Event-Driven Architecture
Loose Coupling: Event-driven architecture promotes loose coupling between services. This separation allows for independent service development, easier maintenance, and seamless scalability.
Asynchronous Communication: Unlike traditional synchronous communication, event-driven systems communicate asynchronously. This asynchronous nature enhances performance and responsiveness as services don't block while waiting for responses.
Scalability: Event-driven systems can scale individual services based on their specific needs, ensuring optimal resource utilization and efficient handling of varying workloads.
Real-time Responsiveness: Event-driven architecture enables real-time updates and notifications. For instance, a chat application can instantly notify users of new messages without constant polling.
Flexibility: The decoupled nature of event-driven systems allows individual services to evolve independently, offering flexibility and agility during development and updates.
2.3: Event Brokers and Publish-Subscribe Model
Event brokers serve as intermediaries between publishers and subscribers. Publishers generate events, while subscribers express interest in specific event types. The publish-subscribe model ensures efficient distribution of events to relevant parties.
2.4: Implementing Event-Driven Architecture
Implementing event-driven architecture involves several key steps:
Event Identification: Identify the meaningful events in your application that warrant asynchronous communication.
Event Schema: Define a common structure for events. JSON is commonly used to ensure consistency across services.
Event Broker Selection: Choose an appropriate event broker that aligns with your requirements. Options include Apache Kafka, RabbitMQ, AWS SNS/SQS, etc.
Publishers: Services generating events publish them to the event broker with relevant metadata.
Subscribers: Services interested in specific events subscribe to those event types. The broker forwards events to subscribers when they occur.
2.5: Node.js and Event-Driven Architecture
Node.js, with its non-blocking and asynchronous nature, is an ideal platform for building event-driven architectures. The built-in EventEmitter class facilitates the creation of custom event-driven patterns within applications.
Node.js Code Sample (Using EventEmitter): javascript Copy code const EventEmitter = require('events'); class OrderService extends EventEmitter { processOrder(order) { // Process order logic this.emit('orderProcessed', order); } } const orderService = new OrderService(); orderService.on('orderProcessed', (order) => { console.log('Order processed:', order); }); orderService.processOrder({ orderId: '123', products: ['item1', 'item2'] });
Section 3: Serverless Computing
Serverless computing abstracts server management, enabling developers to focus solely on code. Functions are executed in response to events, and cloud providers handle scaling and resource allocation.
References:
AWS Lambda: https: aws.amazon.com/lambda/
Azure Functions: https://azure.microsoft.com/en-us/services/functions/
Google Cloud Functions: https://cloud.google.com/functions
Section 4: Building an Event-Driven Serverless Microservices Web App
4.1: Service Architecture
For our practical example, let's design a simplified e-commerce application using microservices: a product catalog service, a user authentication service, and an order processing service.
4.2: Event Broker
Selecting a suitable event broker, such as Apache Kafka or AWS SNS/SQS, is crucial for enabling effective communication between services.
Node.js Code Sample: javascript Copy code const { Kafka } = require('kafkajs'); const kafka = new Kafka({ clientId: 'my-app', brokers: ['kafka1:9092', 'kafka2:9092'], }); const producer = kafka.producer(); await producer.connect(); await producer.send({ topic: 'user_registered', messages: [{ value: JSON.stringify({ userId: '123', name: 'John' }) }], }); await producer.disconnect(); 4.3: Serverless Functions Implement serverless functions for each microservice using AWS Lambda or equivalent serverless platforms. Node.js Code Sample: javascript Copy code exports.handler = async (event) => { const { userId, productId } = JSON.parse(event.body); // Perform authentication and order processing const order = await processOrder(userId, productId); return { statusCode: 200, body: JSON.stringify(order), }; };
Section 5: Deployment and Scalability
Deploying microservices in a serverless environment simplifies scaling. Tools like the Serverless Framework and AWS CDK aid in the deployment process.
References:
Serverless Framework: https://www.serverless.com/framework/docs/
AWS CDK: https://aws.amazon.com/cdk/
Conclusion:
In this comprehensive lecture, we have explored "Event-Driven Serverless Microservices Web Application Engineering." By understanding microservices architecture, the principles of event-driven design, and the capabilities of serverless computing, you're now equipped to design and develop modern web applications that are highly responsive, scalable, and easily maintainable. Remember to refer to the provided references for further exploration and hands-on practice. As you continue your journey, embrace these concepts to stay at the forefront of web application engineering. Your ability to blend these powerful methodologies will enable you to create cutting-edge applications that cater to the evolving needs of the digital landscape.