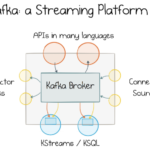
Introduction to Kafka as a Streaming Platform
September 10, 2023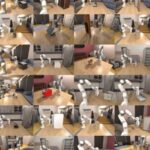
Technical Complexity Analysis of the GenSim2 Framework: Data Augmentation, Proprioceptive Point-Cloud Transformer, and Multimodal Task Planning Calculation
November 1, 2024In the modern business environment, providing efficient, high-quality customer service is essential. Companies face the challenge of managing high volumes of customer queries and support tickets while maintaining fast response times and accuracy. One solution to this challenge is the automation of customer support services using Large Language Models (LLMs), such as GPT-3, BERT, or RoBERTa. These models are powerful, pre-trained systems capable of understanding and generating human-like text. Combined with frameworks like Haystack, businesses can build intelligent, scalable, and domain-specific customer service solutions.
This article presents a comprehensive guide on how developers can fine-tune LLMs and integrate Haystack to create a highly efficient AI-driven customer support system. We will discuss each technical phase in detail, providing insights on data preparation, fine-tuning the model, and integrating Haystack to handle customer queries in real-time without human intervention.
Phase 1: Selecting the Pre-trained Language Model
The first step in building an AI-powered customer support system is choosing an appropriate pre-trained Large Language Model (LLM). Pre-trained models like GPT, BERT, and RoBERTa can be easily integrated into AI systems for natural language understanding (NLU) and natural language generation (NLG) tasks. Platforms like Hugging Face offer an extensive range of pre-trained models that can be used as the starting point for fine-tuning.
These models have already been trained on large amounts of general-purpose data, giving them a broad understanding of language. However, to specialize in a specific domain, such as customer support for a retail business, additional fine-tuning is required. Fine-tuning allows the LLM to become proficient in answering domain-specific queries related to the company's products, services, or industry jargon.
Pre-trained Model Selection Example
Using Hugging Face’s transformers library, developers can easily load a pre-trained model and tokenizer. In this case, let's consider the use of BART (Bidirectional and Auto-Regressive Transformers), which is excellent for tasks like summarization, question-answering, and dialogue systems.
from transformers import AutoModelForSeq2SeqLM, AutoTokenizer # Load pre-trained BART model from Hugging Face model_name = "facebook/bart-large" tokenizer = AutoTokenizer.from_pretrained(model_name) model = AutoModelForSeq2SeqLM.from_pretrained(model_name)
Phase 2: Data Preparation and Preprocessing
Before fine-tuning the selected LLM, the most critical step is preparing the dataset. For customer support, datasets typically include customer support tickets, chat logs, FAQs, product documentation, and historical queries. These datasets should be cleaned and organized to reflect the types of inquiries the AI model will handle.
Key Data Preparation Steps:
- Text Preprocessing: Data must be preprocessed by cleaning up any unnecessary characters, removing irrelevant information, and splitting customer queries into question-answer pairs.
- Tokenization: LLMs process text by breaking it down into tokens. Tokenization converts the text into tokens, allowing the model to interpret and generate text more efficiently.
- Data Labeling: Data should be labeled according to customer intents, such as "order tracking," "refund request," or "technical issues."
In Python, tokenization can be achieved with Hugging Face’s tokenizer utility:
from datasets import load_dataset # Load a sample dataset (SQuAD for this example) dataset = load_dataset("squad") # Tokenize the dataset for fine-tuning def tokenize_function(examples): return tokenizer(examples["context"], truncation=True, padding="max_length") tokenized_datasets = dataset.map (tokenize_function, batched=True)
For customer support, domain-specific data (e.g., transcripts, FAQ) would be used in place of a general-purpose dataset like SQuAD.
Phase 3: Fine-tuning the LLM
Once the data is ready, the next step is to fine-tune the LLM. Fine-tuning is the process of updating a pre-trained model's weights using a domain-specific dataset to make it more specialized in handling the target task. This step is essential in tailoring the model to meet the specific needs of a business.
Fine-tuning Example:
from transformers import Trainer, TrainingArguments # Set training arguments training_args = TrainingArguments( output_dir="./results", evaluation_strategy="epoch", learning_rate=2e-5, per_device_train_batch_size=16, per_device_eval_batch_size=16, num_train_epochs=3, ) # Initialize the Trainer trainer = Trainer( model=model, args=training_args, train_dataset=tokenized_datasets["train"], eval_dataset=tokenized_datasets["validation"], ) # Fine-tune the model trainer.train()
Fine-tuning allows the LLM to learn from the provided dataset, ensuring that it generates more accurate and relevant responses in the context of customer service inquiries. This model will now understand the intricacies of the specific domain it's been fine-tuned for.
Fine-tuning can be done on local machines or using cloud-based services such as AWS EC2, Azure ML, or Google Cloud AI Platform to manage compute-heavy tasks.
Phase 4: Building a Retrieval-Augmented Generation (RAG) Pipeline
While LLMs are good at generating human-like text, they may occasionally generate irrelevant or outdated responses. To ensure accuracy, especially in customer support, integrating a Retrieval-Augmented Generation (RAG) Pipeline is crucial. This method combines LLM generation with a document retrieval system that searches for relevant documents (such as FAQs or product manuals) to provide contextually accurate answers.
Haystack is an excellent open-source framework for building RAG Pipelines. Haystack allows for document storage, retrieval, and the integration of fine-tuned LLMs for question answering.
Setting up Haystack’s Document Store
In this step, developers must set up a document store (e.g., Elasticsearch, FAISS, or SQL-based storage) where the knowledge base, FAQs, or documents are stored. Elasticsearch is widely used in industry for its robustness and scalability.
from haystack.document_stores import FAISSDocumentStore from haystack.nodes import DensePassageRetriever, FARMReader # Set up FAISS Document Store document_store = FAISSDocumentStore (embedding_dim=768, faiss_index_factory_str="Flat") # Initialize DensePassageRetriever retriever = DensePassageRetriever( document_store=document_store, query_embedding_model="facebook/dpr-question_encoder-single-nq-base", passage_embedding_model="facebook/dpr-ctx_encoder-single-nq-base", use_gpu=True, ) # Write documents into the store (assuming documents are pre-processed) document_store.write_documents(documents) document_store.update_embeddings(retriever)
Implementing the Retrieval-Augmented Generation (RAG) Pipeline
Once the documents are indexed in the store, the fine-tuned LLM can be integrated into the Haystack pipeline to process customer queries. The retriever fetches relevant documents, and the LLM generates responses based on the retrieved information.
from haystack.pipelines import ExtractiveQAPipeline # Initialize a reader (fine-tuned LLM) reader = FARMReader(model_name_or_path="facebook/bart-large", use_gpu=True) # Set up the Extractive QA Pipeline pipeline = ExtractiveQAPipeline(reader=reader, retriever=retriever) # Example query query = "How can I track my order?" # Query the pipeline prediction = pipeline.run(query=query, params={"Retriever": {"top_k": 10}}) print(prediction["answers"])
In this example, the customer query about order tracking is handled by Haystack’s pipeline. The system retrieves relevant documents and generates an accurate response using the fine-tuned LLM.
Phase 5: Deploying the AI Model
After fine-tuning the LLM and setting up the RAG pipeline, the next step is to deploy the customer support system in production. This step involves hosting the AI model and making it accessible to end users through APIs, web applications, or chat interfaces.
Cloud platforms such as AWS Lambda, Azure Functions, or Google Cloud Functions offer scalable, serverless options for deploying AI models. You can also use FastAPI to create a REST API that interacts with the Haystack pipeline.
API Deployment with FastAPI
from fastapi import FastAPI app = FastAPI() # Initialize pipeline pipeline = ExtractiveQAPipeline(reader=reader, retriever=retriever) @app.post("/query/") async def query_handler(query: str): result = pipeline.run(query=query) return {"answers": result["answers"]} # Start FastAPI application (run: uvicorn my_app:app --reload)
This deployment approach allows for easy scaling as the number of customer queries increases, ensuring consistent performance and response times.
Phase 6: Monitoring and Continuous Improvement
Deploying the AI-powered customer support system is only the beginning. Continuous monitoring and improvement are necessary to ensure that the model remains effective. Monitoring performance metrics such as response accuracy, query resolution time, and user satisfaction helps identify areas for improvement.
Key Metrics to Monitor:
- Query Resolution Time: Time taken for the AI system to provide a response.
- Accuracy: The percentage of customer queries resolved without needing human intervention.
- Satisfaction Scores: User feedback can be captured through post-interaction surveys or chatbot ratings.
In addition, incorporating a human-in-theLarge Language Models (LLMs) and Haystack for Scalable AI Customer Support Systems**
In today’s digital-first world, businesses are continuously looking for ways to improve their customer service efficiency without sacrificing quality. One promising approach is to harness Large Language Models (LLMs) like GPT-3, BERT, and others to create automated, AI-driven customer support systems. By fine-tuning these models with domain-specific data and integrating them with retrieval frameworks like Haystack, businesses can deliver real-time, accurate, and intelligent responses to customer queries without requiring human intervention.
This article will explore in-depth how developers can implement such an AI customer support system using LLMs and Haystack, from model selection and fine-tuning to deployment and real-time query handling. Additionally, we will provide code examples in Python for key steps, focusing on practical deployment for real-world business use.
Practical Exmaple
1. Pre-trained Model Selection for Customer Support
Choosing the right LLM is the first critical step in creating an AI-powered customer support system. Hugging Face offers various pre-trained models that can be adapted to domain-specific tasks such as answering customer queries, generating summaries, or processing complex FAQs.
Popular models include:
- GPT-3 (Generative Pre-trained Transformer 3): Known for its ability to generate human-like text and handle complex language tasks.
- BERT (Bidirectional Encoder Representations from Transformers): Specialized in understanding the context of words in a sentence, making it ideal for tasks like question answering.
- RoBERTa (Robustly Optimized BERT): An optimized variant of BERT with improved performance.
After selecting a suitable pre-trained model, developers can fine-tune it using specific customer service data, such as FAQs, historical chats, and other relevant documents.
Loading a Pre-trained Model
Here’s an example of how to load a pre-trained BART model using Hugging Face’s transformers library:
from transformers import AutoModelForSeq2SeqLM, AutoTokenizer # Load a pre-trained model and tokenizer model_name = "facebook/bart-large" tokenizer = AutoTokenizer.from_pretrained(model_name) model = AutoModelForSeq2SeqLM.from_pretrained(model_name)
2. Data Preparation for Fine-Tuning
Fine-tuning is essential for adapting a general-purpose LLM to understand domain-specific queries. For customer support, you need to gather relevant datasets, such as:
- FAQ documents
- Chat logs
- Product knowledge bases
Once you have the data, it needs to be cleaned and preprocessed. Key steps include removing irrelevant information, tokenizing text (so the LLM can process it), and labeling data based on customer intents (e.g., tracking orders, requesting refunds).
Example: Tokenizing a Dataset
from datasets import load_dataset # Load dataset (e.g., SQuAD for question-answer tasks) dataset = load_dataset("squad") # Tokenize the dataset def tokenize_function(examples): return tokenizer(examples["context"], truncation=True, padding="max_length") tokenized_datasets = dataset.map(tokenize_function, batched=True)
For customer support tasks, a custom dataset based on business-specific content is necessary.
3. Fine-tuning the Language Model
Once the dataset is ready, fine-tuning the model is the next step. Fine-tuning involves training the model on the specific customer support dataset, which allows the model to generate more accurate, domain-specific responses.
Fine-tuning is usually done using libraries like Hugging Face's transformers, which makes the process straightforward with tools like Trainer for managing the training loop.
Fine-tuning Example
from transformers import Trainer, TrainingArguments # Define training arguments training_args = TrainingArguments( output_dir="./results", evaluation_strategy="epoch", learning_rate=2e-5, per_device_train_batch_size=16, per_device_eval_batch_size=16, num_train_epochs=3, ) # Initialize Trainer trainer = Trainer( model=model, args=training_args, train_dataset=tokenized_datasets["train"], eval_dataset=tokenized_datasets["validation"], ) # Fine-tune the model trainer.train()
Fine-tuning the model ensures it performs well in customer support scenarios, such as answering common questions or assisting with specific requests.
4. Building a Retrieval-Augmented Generation (RAG) Pipeline with Haystack
An important step in building a robust customer support system is to implement a Retrieval-Augmented Generation (RAG) pipeline. This pipeline ensures that responses are accurate by first retrieving relevant documents (e.g., FAQ pages or product manuals) and then generating an answer based on the retrieved information.
Haystack provides the tools for this, enabling developers to:
- Store documents (e.g., FAQs, customer service logs).
- Retrieve relevant documents based on customer queries.
- Generate answers using the fine-tuned LLM.
Setting Up the Haystack Document Store
Haystack supports various document stores, including Elasticsearch and FAISS. Here’s an example of how to set up a FAISS document store and retrieve documents using DensePassageRetriever.
from haystack.document_stores import FAISSDocumentStore from haystack.nodes import DensePassageRetriever # Initialize FAISS document store document_store = FAISSDocumentStore(embedding_dim=768, faiss_index_factory_str="Flat") # Initialize retriever retriever = DensePassageRetriever( document_store=document_store, query_embedding_model="facebook/dpr-question_encoder-single-nq-base", passage_embedding_model="facebook/dpr-ctx_encoder-single-nq-base", use_gpu=True, ) # Write documents to the store document_store.write_documents(documents) document_store.update_embeddings(retriever)
Implementing the Retrieval-Augmented Generation Pipeline
Now that the documents are indexed, you can implement a pipeline that uses the retriever to fetch relevant documents and the fine-tuned LLM (reader) to generate answers.
from haystack.pipelines import ExtractiveQAPipeline # Initialize the reader (fine-tuned model) reader = FARMReader(model_name_or_path="facebook/bart-large", use_gpu=True) # Create an Extractive QA Pipeline pipeline = ExtractiveQAPipeline(reader=reader, retriever=retriever) # Example query query = "How can I reset my password?" # Run the pipeline prediction = pipeline.run(query=query, params={"Retriever": {"top_k": 10}}) print(prediction["answers"])
This setup retrieves the most relevant documents, and the fine-tuned LLM processes these documents to generate a customer-facing response.
5. Deploying the Model with FastAPI
After building and fine-tuning the model, the next step is deploying it in a real-world scenario. One option is to create a REST API using FastAPI, which interacts with the Haystack pipeline to process customer queries.
Deployment Example with FastAPI
from fastapi import FastAPI from haystack.pipelines import ExtractiveQAPipeline app = FastAPI() # Initialize the pipeline pipeline = ExtractiveQAPipeline(reader=reader, retriever=retriever) @app.post("/query/") async def query_handler(query: str): # Process the query with the pipeline result = pipeline.run(query=query) return {"answers": result["answers"]} # Run FastAPI app locally (use command: uvicorn my_app:app --reload)
This setup allows the AI customer support system to be accessed via a simple API. As users submit queries, the system fetches relevant documents and generates accurate responses in real-time.
6. Monitoring and Continuous Improvement
To ensure long-term success, monitoring the performance of the AI system is critical. Continuous learning is essential as customer interactions evolve, requiring the model to adapt over time.
Metrics to Monitor:
- Response Accuracy: Measure the correctness of responses based on customer feedback.
- Response Time: Ensure the system responds in a timely manner.
- Satisfaction Score: Track how satisfied customers are with the AI’s responses.
Regularly fine-tune the model with new customer data and update the document store with fresh knowledge bases and FAQs to improve performance.
Conclusion
Fine-tuning LLMs and integrating them with Haystack’s retrieval capabilities offers a powerful solution for automating customer support at scale. By combining document retrieval with generative models, companies can deliver efficient and accurate responses to customer queries without the need for human intervention. As shown in the provided code examples, developers can easily implement this system using modern Python libraries, making AI-driven customer service an accessible and valuable asset for businesses.
For further reading and resources: