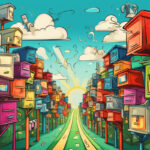
Event-Driven Serverless Microservices; Web Application Engineering
August 4, 2023Introduction
In modern software engineering, designing systems that are scalable, maintainable, and capable of handling complex business requirements is paramount. Two architectural patterns that have gained significant traction in achieving these goals are Event Sourcing and Event-Driven Architecture (EDA). While they are often discussed in tandem, it's crucial to understand their distinct roles and how they can complement each other to build robust software systems.
This article delves into the technical intricacies of Event Sourcing and Event-Driven Architecture, exploring their principles, advantages, and practical applications. We'll provide code examples in C#, TypeScript, and C++ to illustrate how these patterns can be implemented across different programming languages.
Event-Driven Architecture
Overview
Event-Driven Architecture is a software design paradigm where the flow of the program is determined by events—signals or messages that indicate that something has happened. This architecture decouples the event producers from event consumers, allowing for a highly scalable and flexible system.
Key Components
- Event Producers: Components that generate events when a significant action occurs.
- Event Consumers: Components that listen for events and react accordingly.
- Event Bus or Broker: A middleware that routes events from producers to consumers.
Benefits
- Scalability: Components can scale independently.
- Flexibility: Easy to add or modify consumers without impacting producers.
- Asynchronous Processing: Enhances performance by handling tasks asynchronously.
Implementation Example
C#: Simple Event Publisher and Subscriber
// Event Publisher public class OrderService { public delegate void OrderPlacedHandler(object sender, OrderEventArgs e); public event OrderPlacedHandler OrderPlaced; public void PlaceOrder(Order order) { // Logic to place order // ... // Raise event OnOrderPlaced(order); } protected virtual void OnOrderPlaced(Order order) { OrderPlaced?.Invoke(this, new OrderEventArgs { Order = order }); } } // Event Subscriber public class NotificationService { public void OnOrderPlaced(object sender, OrderEventArgs e) { // Send notification Console.WriteLine($"Notification sent for Order ID: {e.Order.Id}"); } } // Usage var orderService = new OrderService(); var notificationService = new NotificationService(); orderService.OrderPlaced += notificationService.OnOrderPlaced; orderService.PlaceOrder(new Order { Id = 1, ProductName = "Laptop" });
Event Sourcing
Overview
Event Sourcing is a pattern where changes to an application's state are stored as a sequence of events. Instead of persisting the current state, the system records each state-changing event. The current state can be reconstructed by replaying these events.
Key Concepts
- Event Store: A database that persists events in order.
- Aggregate: A cluster of domain objects treated as a single unit.
- Command: An instruction to perform an action that results in an event.
Benefits
- Auditability: Complete history of changes is maintained.
- Temporal Querying: Ability to reconstruct past states.
- Decoupling: Commands and queries are separated, often implemented with CQRS (Command Query Responsibility Segregation).
Implementation Example
TypeScript: Simple Event Sourcing for Account Balance
// Event Interfaces interface Event { type: string; data: any; timestamp: Date; } interface DepositEvent extends Event { type: 'Deposit'; data: { amount: number }; } interface WithdrawEvent extends Event { type: 'Withdraw'; data: { amount: number }; } // Event Store class EventStore { private events: Event[] = []; public addEvent(event: Event): void { this.events.push(event); } public getEvents(): Event[] { return this.events; } } // Account Aggregate class Account { private balance: number = 0; constructor(private eventStore: EventStore) {} public deposit(amount: number): void { const event: DepositEvent = { type: 'Deposit', data: { amount }, timestamp: new Date(), }; this.eventStore.addEvent(event); this.apply(event); } public withdraw(amount: number): void { const event: WithdrawEvent = { type: 'Withdraw', data: { amount }, timestamp: new Date(), }; this.eventStore.addEvent(event); this.apply(event); } private apply(event: Event): void { switch (event.type) { case 'Deposit': this.balance += event.data.amount; break; case 'Withdraw': this.balance -= event.data.amount; break; } } public getBalance(): number { return this.balance; } public replay(): void { this.balance = 0; // Reset balance const events = this.eventStore.getEvents(); for (const event of events) { this.apply(event); } } } // Usage const eventStore = new EventStore(); const account = new Account(eventStore); account.deposit(100); account.withdraw(50); console.log(`Current Balance: ${account.getBalance()}`); // Output: 50 // Replaying events account.replay(); console.log(`Balance after replay: ${account.getBalance()}`); // Output: 50
Event Sourcing vs. Event-Driven Architecture
While both patterns deal with events, they serve different purposes and operate at different layers within a system.
Event-Driven Architecture
- Focus: Communication between decoupled components.
- Events: Notifications that something has happened, often used to trigger actions in other parts of the system.
- Use Case: Real-time processing, microservices communication, and asynchronous workflows.
Event Sourcing
- Focus: State management and persistence.
- Events: Immutable records that represent state changes.
- Use Case: Systems requiring audit logs, temporal queries, and complex state management.
Complementary Usage
Event Sourcing can be implemented within an Event-Driven Architecture to persist events that are also used to trigger other processes. This combination allows for robust state management along with scalable and decoupled component interaction.
Pros and Cons
Event-Driven Architecture
Pros
- High Throughput: Efficient handling of a large number of events.
- Low Latency: Quick response times due to asynchronous processing.
- Scalability: Components can scale horizontally.
Cons
- Complexity: Debugging and tracing can be challenging.
- Consistency: Ensuring data consistency across components may require additional mechanisms.
Event Sourcing
Pros
- Auditability: Full history of state changes is maintained.
- Flexibility: Ability to reconstruct and analyze past states.
- Resilience: Easier recovery from failures by replaying events.
Cons
- Complexity: Requires careful design of event schemas and handling.
- Storage Overhead: Event stores can grow large over time.
- Performance: Replaying events can be time-consuming without snapshots.
Practical Applications
Uses of Event-Driven Architecture
- Microservices Communication: Decoupling services in a microservices architecture.
- Real-Time Systems: Trading platforms, IoT devices, and gaming applications.
- Reactive Systems: Applications that react to user interactions or external events promptly.
Implementation Example
C++: Event-Driven GUI Application
#include <iostream> #include <functional> #include <unordered_map> #include <vector> class EventEmitter { public: using Listener = std::function<void(int)>; void on(const std::string& event, Listener listener) { listeners[event].push_back(listener); } void emit(const std::string& event, int data) { if (listeners.count(event)) { for (auto& listener : listeners[event]) { listener(data); } } } private: std::unordered_map<std::string, std::vector<Listener>> listeners; }; // Usage int main() { EventEmitter emitter; emitter.on("button_click", [](int buttonId) { std::cout << "Button " << buttonId << " clicked." << std::endl; }); // Simulate button click emitter.emit("button_click", 1); return 0; }
Uses of Event Sourcing
- Financial Systems: Banking applications where transaction history is critical.
- Audit Trails: Compliance requirements necessitating detailed logs.
- Complex Domain Logic: Systems where state changes are complex and interdependent.
Implementation Example
C#: Event Sourcing with Snapshots
// Event Base Class public abstract class Event { public Guid Id { get; } = Guid.NewGuid(); public DateTime Timestamp { get; } = DateTime.UtcNow; } // Specific Events public class ProductAddedEvent : Event { public string ProductName { get; set; } public int Quantity { get; set; } } public class ProductRemovedEvent : Event { public string ProductName { get; set; } } // Event Store with Snapshotting public class EventStore { private readonly List<Event> _events = new List<Event>(); private Dictionary<string, object> _snapshot = new Dictionary<string, object>(); public void AddEvent(Event @event) { _events.Add(@event); // Snapshot logic if (_events.Count % 10 == 0) { TakeSnapshot(); } } public IEnumerable<Event> GetEvents() => _events; private void TakeSnapshot() { // Logic to create a snapshot of the current state // ... Console.WriteLine("Snapshot taken."); } } // Usage var store = new EventStore(); store.AddEvent(new ProductAddedEvent { ProductName = "Book", Quantity = 10 }); store.AddEvent(new ProductRemovedEvent { ProductName = "Book" }); // Add more events...
Combining Event Sourcing and Event-Driven Architecture
By integrating Event Sourcing within an Event-Driven Architecture, systems can achieve both robust state management and flexible inter-component communication.
Benefits of Combining
- Unified Events: Events serve both as state changers and triggers for other processes.
- Consistency: Single source of truth for state changes.
- Simplified Architecture: Reduced need for separate messaging infrastructure.
Implementation Considerations
- Event Design: Events must be designed to serve both purposes effectively.
- Infrastructure: Requires an event store and an event bus or broker.
- Performance Optimization: Implement snapshots or caching to mitigate replay overhead.
Conclusion
Event Sourcing and Event-Driven Architecture are powerful patterns that address different aspects of system design. Event-Driven Architecture focuses on decoupling and asynchronous communication between components, while Event Sourcing deals with state persistence and auditability. When used together, they provide a comprehensive approach to building scalable, maintainable, and robust software systems.
Understanding the distinctions and synergies between these patterns allows architects and developers to make informed decisions, leveraging the strengths of each to meet specific business and technical requirements.
References
- Domain-Driven Design by Eric Evans
- Enterprise Integration Patterns by Gregor Hohpe and Bobby Woolf
- Event Sourcing by Martin Fowler